You may have noticed that your first signal processing pipeline does not actually process any data...
In this part of the tutorial, we are going to fix this. We are also going to generate a multi-channel signal and then store it in the MCsweep object.
The great advantage of this is that we can use the signal processing capabilities built into MCsweep together with other pipeline plugins already developed for working with MCsweep objects. E.g. we don't actually need to develop our own plotter plugin because it is already there.
To move from single to multichannel signals we can again use the SignalGenerator class to generate multichannel signals. And methods like makeNChannelSineWave(duration,frequency,amplitude, theta, sampleRate, numChannels) will do that for us.
We are now going to build our signal processing pipeline step by step. And we start again with a simple signal pipeline. Only this time, we are building it from already available plugins instead of developing them ourselves.
import RZ2ephys def example8(): aSignalGenerator = pipeline.SignalGenerator() duration = 1 frequency = 1.0 amplitude = 1.0 theta = 0.0 sampleRate = 24414 numChannels = 32 aDatasource = pipeline.Datasource() aDatasource.setName("Our Signal Datasource") aDisplay = pipeline.MplSweepDataDisplay(plt) aDisplay.setName("Our Signal DataDisplay") aDatasink = pipeline.Datasink() aDatasink.setName("Our Signal Datasink") aDatasource.setOutput(aDisplay) aDisplay.setOutput(aDatasink) for n in range(100): #generate multichannel data time, signalBuffer = aSignalGenerator.makeNChannelSineWave(duration,frequency,amplitude, theta, sampleRate, numChannels) lenOfSignal = len(signalBuffer[0:,0]) sigChunk=np.zeros(lenOfSignal*numChannels) sigTupel = tuple(sigChunk) #create MCsweep object data=RZ2ephys.MCsweep(sigTupel,lenOfSignal,sampleRate,0) for chanIdx in range(numChannels): data.signal[0:,chanIdx] = signalBuffer[0:,chanIdx]*np.random.random(1)#amplitude modulation aDataframe = pipeline.Dataframe() aDataframe.setFrameNumber(n) aDataframe.setData(data) aDatasource.addDataframe(aDataframe) aDatasource.run()
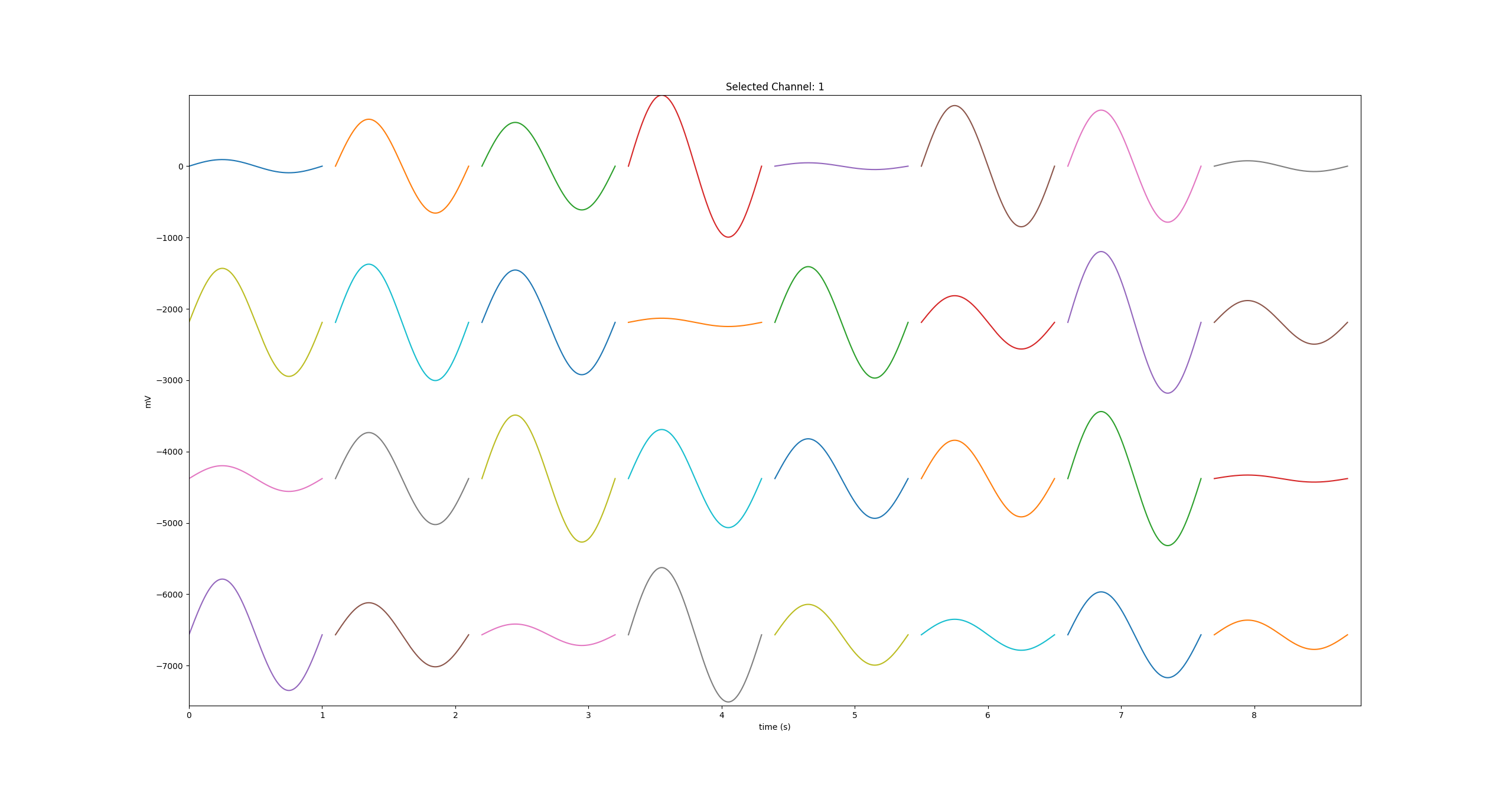
The RZ2ephys.MCsweep is a very important component with a lot of very useful methods.