We are now going to add the blanking pipeline to remove the blanking artifacts. Apart from the additional plugin and additional parameters, the code is identical to example15.
The new parameter blankingEndOffsetInSeconds is set via
aSweepInterBlankingPlugin.setEndOffsetInSeconds(blankingEndOffsetInSeconds)
and allows extending the blanking duration by some fixed value in seconds. This may be needed because after filtering the artifacts, the segment contaminated with artifacts may extend beyond the original stimulation duration. To make sure that all artifacts are properly blanked and don't affect the automatic scaling of the plot, we simply add a few milliseconds to the actual stimulation duration.
After making the modification, this is what the code looks like:
def example16(): sampleRate = 24414 numChannels = 32 sweepLen = int(sampleRate/2) enableCISimulation = True enableBlanking = True #set up the stimulation parameter object #set stimulation parameters level_dB=5 # in dB in relation to 100 uA clickRate=50 # 300, 900, 1800 ears='left' # other options are 'right' and 'both' Must be set to 'left' or 'right' during simulation duration = 0.01 # This defines the duration of the stimulation numClicks = 1 nloop = 0 reference_Simulation=0.100 # set reference to 100 uA levelRePeak = True # normally we set levels relative to RMS If you want to set them relative to abs max instead, set this to True clickShape_CI_Simulation = np.array([0,1,0,-1,0]) # use a biphasic pulse blankingEndOffsetInSeconds = 0.012 # This offset shifts the end of the blanking. This can be useful if the filter artifacts occur beyond the actual duration of the stimulation. #set up the stimulation parameter object stimulatorModule.soundPlayer=stimulatorModule.pygameSoundHardware() stimulatorModule.soundPlayer.sampleRate = 25000 stim=stimulatorModule.clickTrainObject() stim.clickShape= clickShape_CI_Simulation stim.stimParams['duration (s)']=duration # this parameter controls the duration of the stimulation. stim.stimParams['ABL (dB)']=level_dB #ABL means Average Binaural Level stim.stimParams['clickRate (Hz)']=clickRate stim.stimParams['Nloop']=nloop stim.stimParams['numClicks']=numClicks stim.reference=reference_Simulation stim.clickShape=clickShape_CI_Simulation stim.levelRePeak=levelRePeak stim.ears=ears stim.ready() aDatasource = pipeline.Datasource() aDatasource.setName("Eyphs PushDataSource") sweepSpikeFiltFiltPlugin = pipeline.SweepSpikeFiltFiltPlugin() sweepSpikeFiltFiltPlugin.setName("Eyphs SweepSpikeFiltFiltPlugin") aSpikeSimulationPlugin = pipeline.SpikeSimulationPlugin() aSpikeSimulationPlugin.setName("Eyphs SpikeSimulationPlugin") aSpikeSimulationPlugin.loadSpikeTemplates("FilteredSpikeTemplates.npy") debugSpikeAmplitudes = [0.0001, 0.0001, 0.0001, 0.0001] debugSpikePositions = [0.1, 0.2, 0.3, 0.4] aSpikeSimulationPlugin.setDebug(False) aSpikeSimulationPlugin.setDebugSpikeAmplitudes(debugSpikeAmplitudes) aSpikeSimulationPlugin.setDebugSpikePositions(debugSpikePositions) aSweepInterBlankingPlugin = pipeline.SweepInterBlankingPlugin() aSweepInterBlankingPlugin.setName("Eyphs SweepInterBlankingPlugin") aSweepInterBlankingPlugin.setEndOffsetInSeconds(blankingEndOffsetInSeconds) aSweepInterBlankingPlugin.setEnabled(enableBlanking) aArtifactSimulationPlugin = pipeline.ArtifactSimulationPlugin() aArtifactSimulationPlugin.setName("Eyphs ArtifactSimulationPlugin") aArtifactSimulationPlugin.setEnabled(enableCISimulation) aSpikeSimulationPlugin = pipeline.SpikeSimulationPlugin() aDisplay = pipeline.MplSweepDataDisplay(plt) aDatasink = pipeline.Datasink() aDatasink.setName("Eyphs DataSink") #assemble the pipeline aDatasource.setOutput(sweepSpikeFiltFiltPlugin) sweepSpikeFiltFiltPlugin.setOutput(aSpikeSimulationPlugin) aSpikeSimulationPlugin.setOutput(aArtifactSimulationPlugin) aArtifactSimulationPlugin.setOutput(aSweepInterBlankingPlugin) aSweepInterBlankingPlugin.setOutput(aDisplay) aDisplay.setOutput(aDatasink) for n in range(100): #generate multichannel data and random background noise sigTupel=tuple(0.000015*np.random.normal(0,1.0, numChannels*sweepLen)) data=RZ2ephys.MCsweep(sigTupel,sweepLen,sampleRate,0) stimObjectCopy = copy.deepcopy(stim) aDataframe = pipeline.Dataframe() aDataframe.setStimObject(stimObjectCopy) aDataframe.setFrameNumber(n) aDataframe.setData(data) aDatasource.addDataframe(aDataframe) aDatasource.run()
And this what the output looks like:
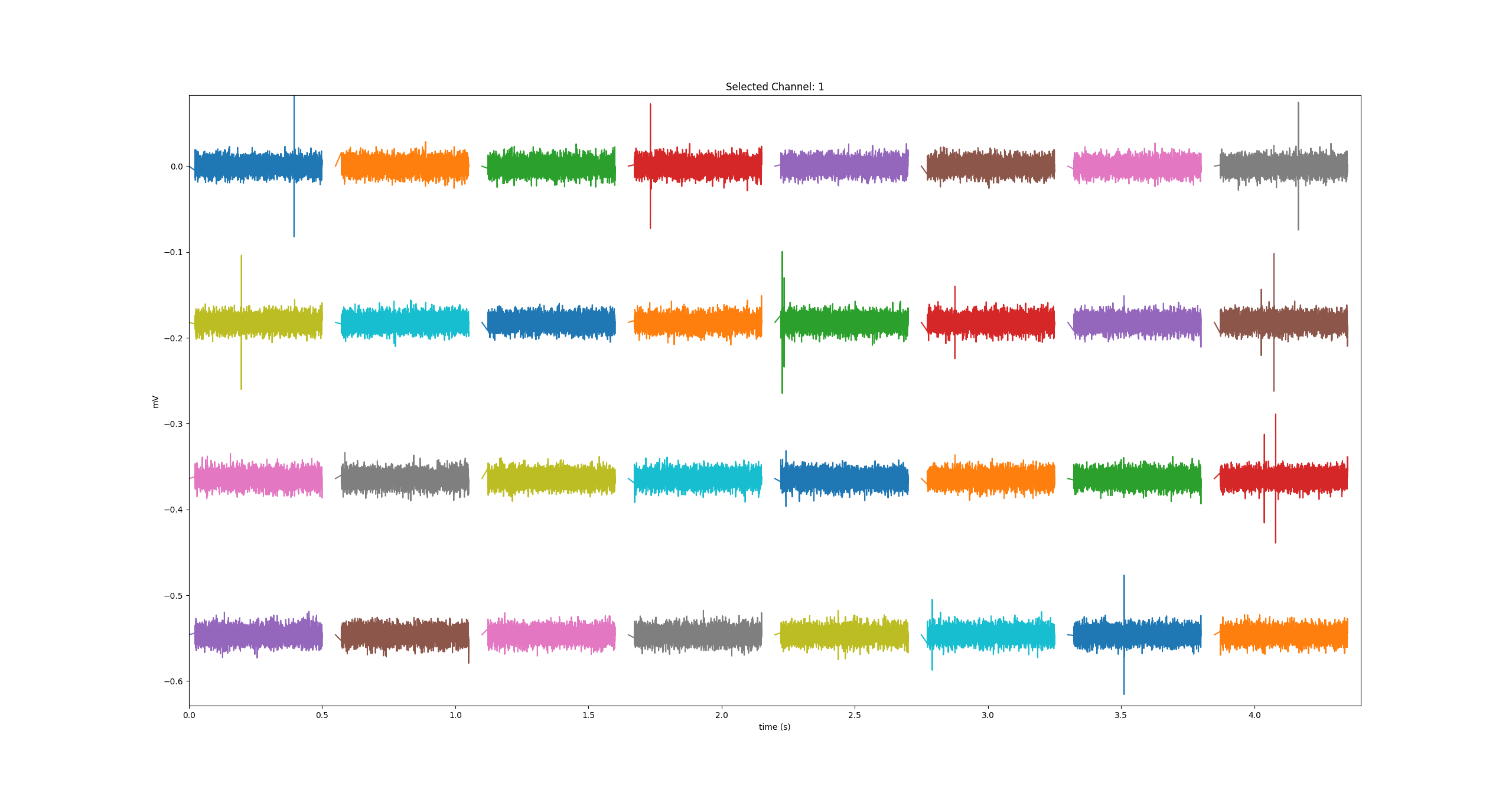
The plot looks almost identical to what we have seen in Fig 24. The result of the blanking can be seen in Fig 27.
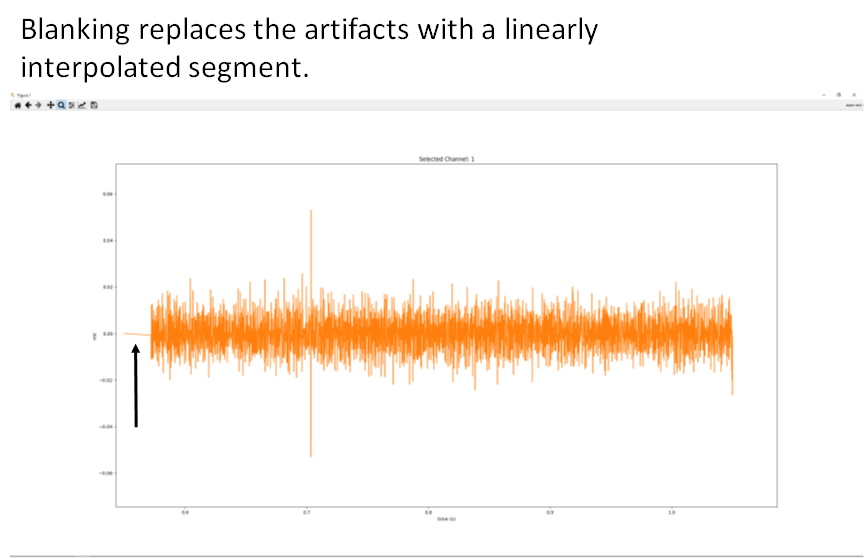